ManageIQ has well-written documentation on working with the REST API. Everything else is either very poorly documented or not documented at all. The only reliable source of information remains the source code. Today we will examine the network configuration when creating virtual machines: adding additional interfaces and configuring them.
Automating the creation of a virtual machine in ManageIQ involves a request (miq_provision_request object) and a task (miq_provision object). The input parameters of the new deployment will be added to the miq_provision_request object as an options hash. This hash will then be passed to the task (or tasks) object, gradually filling it with additional parameters. The options hash may differ slightly depending on the type of OS of the ordered VM and the target virtualization platform. In my case, it will be a Linux guest OS on the VMware platform.
Setting up virtual machines, not only their network parameters, comes down to filling the options hash with certain elements – <key:value> pairs. The following keys are used for network settings: :addr_mode, :ip_addr, :gateway, :subnet_mask, :mac_address, :dns_servers, :dns_suffixes, :network_adapters, :networks, :nic_settings, :vlan… Some parameters are added automatically with a default value. The list of parameters, their valid values and default values, can be found in the descriptions of the virtual machine preparation dialogs: /Automation/Automate/Customization/Provisioning Dialogs/*.
The miq_provision object also has several auxiliary methods designed to simplify the configuration process: set_vlan, set_dvs, set_network_address_mode, set_network_adapter, set_nic_settings
In addition, there are parameters and methods specific to cloud providers, such as: floating_ip_address, cloud_network, cloud_subnet. In this article, working with cloud providers will not be considered.
One network adapter
To configure a VM with one network interface, the following parameters/values are used:
- Number of interfaces: :network_adapters – already added and set to [1, ‘1’] by default;
- Connected network: :vlan – port group name, if the port group is on DVS, then ‘dvs_*’ should be added to the name. You can use the
miq_request.set_vlan('pg_web_app')
ormiq_request.set_dvs('pg_web_app')
methods. - Addressing type: :addr_mode, ‘dhcp’ (default value) or ‘static’,
miq_request.set_network_address_mode('static')
method;- Additionally, for static addressing, you will need to specify:
- IP address: :ip_addr;
- Network mask: :subnet_mask;
- Gateway: :gateway;
- DNS servers: :dns_servers.
- Additionally, for static addressing, you will need to specify:
- + Additional OS-specific network parameters (if necessary).
miq_request = $evm.root['miq_provision_request']
miq_request.set_option(:vlan, ['pg_web_app', 'pg_web_app'])
# or you can use a method that is completely identical to the previous entry:
# miq_request.set_vlan('pg_web_app')
miq_request.set_option(:addr_mode, ['static', 'Static'])
# miq_request.set_network_address_mode('static')
miq_request.set_option(:ip_addr,'192.168.111.7')
miq_request.set_option(:gateway,'192.168.111.1')
miq_request.set_option(:subnet_mask,'255.255.255.0')
miq_request.set_option(:dns_servers,'192.168.101.2, 192.168.102.2')
You can read a specific value from the options hash like this: miq_request.get_option(:ip_addr)
. Note that if the hash contains an array, the get_option method will return only the first element of this array. This is convenient when getting properties such as addr_mode or vlan, but if you need the entire array, it is better to do this: miq_request.options.fetch(:nic_settings, [])
Multiple network adapters
Additional network interfaces are configured via two special parameters: :networks and :nic_settings, which accept arrays of properties. Two additional methods (on the miq_provision object): set_network_adapter and set_nic_settings, simplify their addition to the hash:
# add an additional network interface
# connect it to the pg_web_app portgroup and set a static IP
index = 1
miq_provision.set_network_adapter(index,
{
:network => 'pg_web_app',
:devicetype => 'VirtualVmxnet3',
:is_dvs => true
})
miq_provision.set_nic_settings(index,
{
:ip_addr => '192.168.111.7',
:subnet_mask => '255.255.255.0',
:addr_mode => ['static', 'Static']
})
These methods can also be used to configure the main network interface by passing 0 (zero) as the value of the first attribute (network interface number). The set_nic_settings method for interface 0 will not add the :nic_settings array to options , it will sequentially add all key/value pairs to the root hash.
By analogy with adding disks, you can create network interfaces using the input parameters of the dialogs in / ManageIQ / Infrastructure / VM / Provisioning / StateMachines / Methods / vmware_PreProvision:
def initialize(handle = $evm)
...
@set_nics = true
end
...
def input_fields_data(prefix)
inputs = prov.options.merge(prov.get_option(:ws_values) || {})
inputs.filter { |key, _value| key.start_with?(prefix) }
.map { |key, value| [key.to_s.scan(/\d+/).first.to_i, value] }
.sort.to_h.values
end
def nic_config(network)
{ network: network,
devicetype: 'VirtualVmxnet3',
is_dvs: true,
connectable: { startconnected: true, allowguestcontrol: true, connected: true } }
end
def set_nics
log(:info, "Processing set_nics...", true)
nics = input_fields_data("add_nic").map { |net| nic_config(net) }
prov.set_option(:networks, nics) if nics.any?
log(:info, "Provisioning object <:networks> updated with <#{nics}>") if nics.any?
log(:info, "Processing set_nics...Complete", true)
end
...
def process_customization
...
set_nics if @set_nics
end
This example searches for all dialog box fields that match the mask ‘add_nicX’. The values of these fields are used to form an array of network interface parameters nics, which is added to the options hash. Addressing can be configured in a similar way, but it is more correct to do this in the integration processes with IPAM.
ManageIQ integration with IPAM
Integrating the VM creation process with external systems is very easy: classes, methods, instances for connecting and exchanging data with the external system are created separately, and then a link to the instance is added as a new stage in the deployment process:
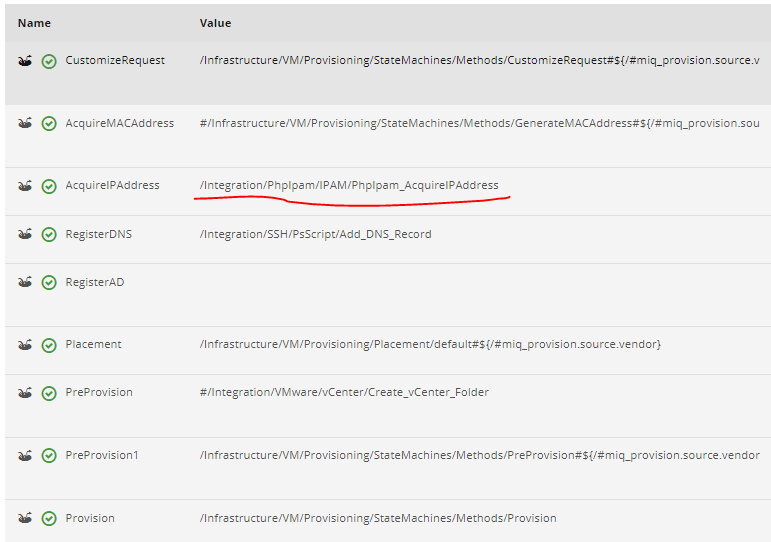
Inside the dedicated PhpIpam_AcquireIPAddress method, you can read the required values from the options hash, find the required subnet, reserve an address in it, and add it to the same hash:
...
nic1_options = {
ip_addr: ip_addr1,
subnet_mask: subnet_mask1,
gateway: getFirstAddress(subnet_mask1, ip_addr1),
dns_domain: subnets1[0]["nameservers"]["namesrv1"],
dns_suffixes: subnets1[0]["nameservers"]["description"],
linux_domain_name: subnets1[0]["nameservers"]["description"]
dns_servers: "#{dns1}, #{dns2}"
}
@prov.set_option(:nic_settings, [nic1_options])
Or the same for two network interfaces:
...
nic1_options = {
ip_addr: ip_addr1,
subnet_mask: subnet_mask1,
gateway: getFirstAddress(subnet_mask1, ip_addr1),
dns_domain: subnets1[0]["nameservers"]["namesrv1"],
dns_suffixes: subnets1[0]["nameservers"]["description"],
linux_domain_name: subnets1[0]["nameservers"]["description"]
dns_servers: "#{dns1}, #{dns2}"
}
nic2_options = {
ip_addr: ip_addr2,
subnet_mask: subnet_mask2,
gateway: "0.0.0.0"
}
@prov.set_option(:nic_settings, [nic1_options, nic2_options])